Thanks for visiting my blog!
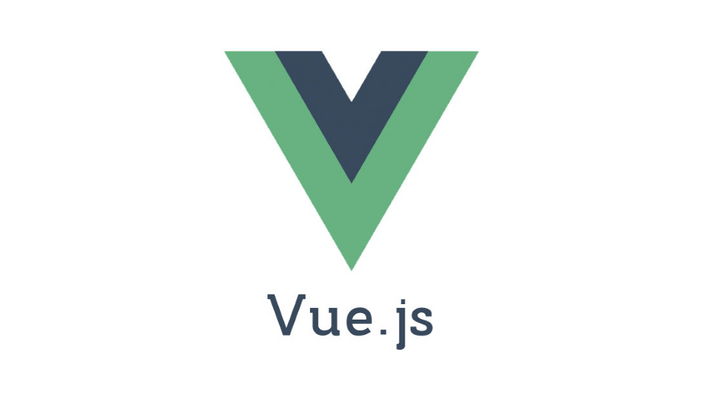
As Vue 3 continues it’s relentless Beta drive (with almost daily Beta builds), all of us Vue developers have to get ready for changes. The one I want to mention today is the changes in mounting a new Vue object.
In prior versions, Vue used the global Vue object to specify things like plugins. In Vue 3, this changes to allow you to mount separate Vue instances with different plugins. Let me show you how.
What is Global Mounting
The idea of starting up a Vue project takes a couple of forms. For example, in Vue 2 you could either just use the JavaScript file or use a transpiler. The startup for both of these cases were similar (but not the same). For example:
Vue.use(VeeValidate);
new Vue({
el: "#index-page",
data: {
name: "Shawn"
}
});
In this simple case, registering the plugin (e.g. VeeValidate) is global and then a new instance of the Vue object can use the validation library. This works great if there is one and only one large Vue object. The same is the case when using transpilers:
import Vue from 'vue';
import router from "./router";
import VeeValidate from "vee-validate";
import store from "./dataStore";
Vue.use(VeeValidate);
new Vue({
store,
router,
}).$mount("#index-page");
Note that no matter how many vue objects are created, they’d still all use the global configuration.
How Vue 3 Changes Global Mounting
In Vue 3, the goal is to better encapsulate plugins and not use a global representation. To do this, you will use the createApp function:
Vue.createApp({
setup() {
return {
name: "Shawn"
}
},
template: `<div>{{ name }}</div>`
})
.use(VeeValidate)
.mount("#theApp");
Notice the plug-in is part of the app object (e.g. use function), not global. If you’re using a transpiler, it becomes clearer what is happening:
import { createApp } from 'vue';
import App from './App.vue'
import VeeValidate from 'VeeValidate';
import router from './router'
import store from './store'
createApp(App)
.use(VeeValidate)
.use(store)
.use(router)
.mount('#app')
Notice that Vuex and the router are also just another plugin. No more magic different ways to inject functionality into your vue object.
What do you think?
