Thanks for visiting my blog!
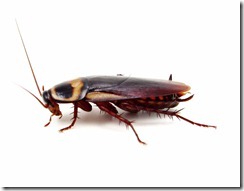
Another day in JavaScript land for this C# guy. I am reading the excellent Professional JavaScript for Web Developers and I am finding more and more that separates the two languages. I know everyone will mention it, but I did read JavaScript: The Good Parts and I liked it but it isn’t as comprehensive as this book.
This time I ran into the fact that JavaScript scopes are handled very different from what C# developers should expect. In C#, we have block scoping. What this means is that any block (e.g. {}) defines a variable scope so that outer scopes can’t see the variables created inside. For example:
// C#
var a = "Hello";
if (true)
{
// This works because there isn't a scope
var b = a;
}
// This doesn't work because the outer scope can't see the inner scope.
var c = b;
In C#, blocks create scopes (e.g. if, for, etc.). In JavaScript scope is actually handled at the ‘context’ level. In JavaScript, a ‘context’ is created by functions, with and try-catch blocks:
// JavaScript
function foo() {
var a = "Hello";
if (true) {
// This works because the outer scope can see the inner scope
var b = a;
}
// This works because ‘if’ doesn’t create a
// context so the b is created in the context.
var c = b;
}
// But Functions create scope so these
// aren't available outside the function
var d = a;
Being aware of these key differences in the scoping between C# and JavaScript will help you not get confused.
For me, I want to know the language (not use another language and generate JavaScript. As a developer, the more languages I understand, the better I am. Trying to fit everything into the C# world, just limits my knowledge, but that’s my opinion. I could be wrong ;)
