Thanks for visiting my blog!
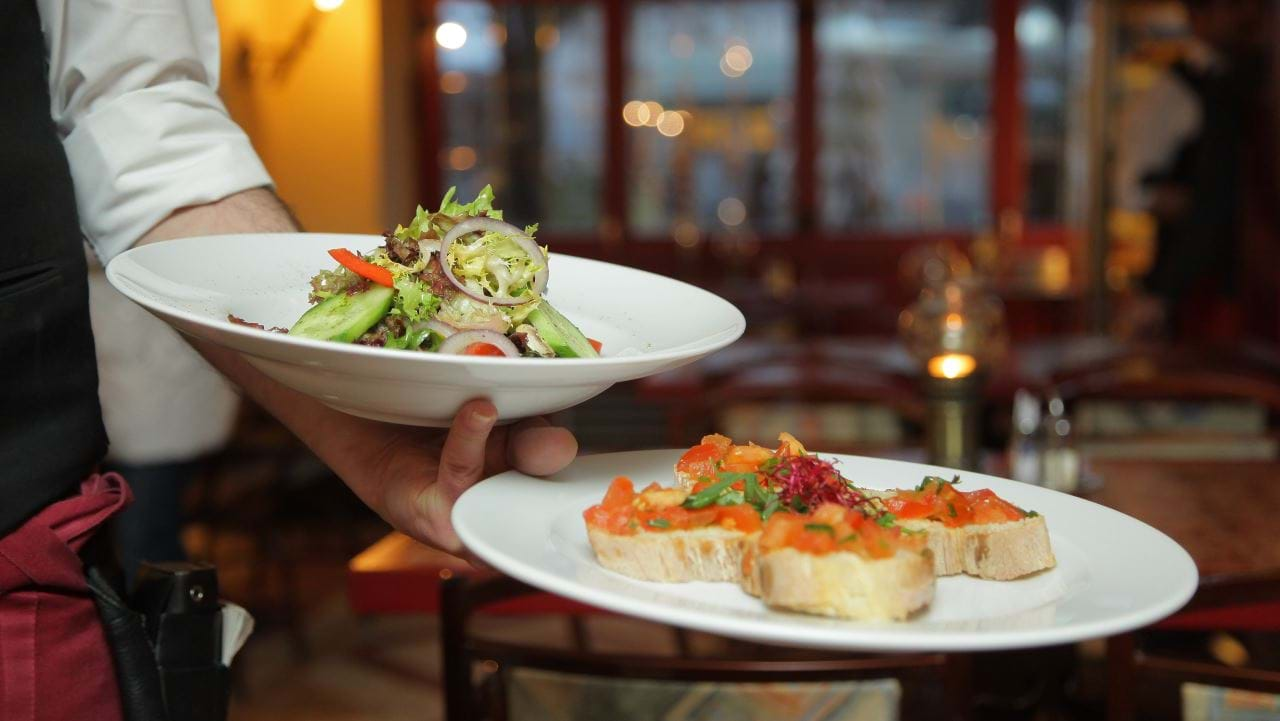
Running a build for your Vue is a common task for many Vue developers. The team behind Vue has been working on a development server to simplify this. This project is called Vite.
I’ve been playing with Vite a little bit and while there are tons of demos showing how to get started, I wanted to show you how to use Vite on your existing projects.
What is Vite?
First of all, Vite is not limited to it’s use with Vue. Vite works with React, Vanilla JS, and Vue. The goal is to allow you to develop modern JavaScript projects without having to do bundling builds on every change.
It’s effectively a development server for JavaScript. It uses addins for different development projects (including Vue of course). You can read the walk-through on the development website:
Adding Vite to Vue CLI Projects
If you’re already using the CLI, adding Vite shouldn’t be that much trouble. The first step is to add Vite and the Vue plugin into your devDependencies:
> npm install vite @vitejs/plugin-vue --save-dev
Once that is complete, you’ll need a vite.config.js (or .ts) file. In that, you’ll specify the plugin (vue in our case):
// vite.config.js
import vue from '@vitejs/plugin-vue'
export default {
plugins: [vue()]
}
Once you have that in place, you’ll need an page to host it. Remember, that you’re going to be using the root of your project as the root of a development web server, so putting an index.html in the project works:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<link rel="icon" href="/favicon.ico" />
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Your App</title>
</head>
<body>
<div id="app"></div>
<script type="module" src="/src/main.js"></script>
</body>
</html>
This is different from your public/index.html that the Vue CLI uses (as it doesn’t need to inject anything), though you can use that as the basis of this file.
Notice that the script tag is pointing at the starting point of the ‘vue’ project. If you have multiple starting points, you’ll need more than one HTML file and since it’s just a web server, that’s just fine.
Next, you’ll need to add vite to the scripts in package.json (here called ‘dev’):
"scripts": {
"serve": "vue-cli-service serve",
"build": "vue-cli-service build",
"lint": "vue-cli-service lint",
"dev": "vite"
},
If you open a console to the project and type:
> npm run dev
You should see it prep and surface your project at an URL.
Vite dev server running at:
> Network: http://172.26.240.1:3000/
> Network: http://192.168.160.1:3000/
> Network: http://172.31.105.19:3000/
> Local: http://localhost:3000/
ready in 369ms.
As you make changes, it will hot-swap code to help you debug in real time.
Let me know if this helps!
