Thanks for visiting my blog!
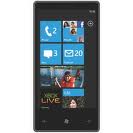
Url: http://wilderminds.blob.core.windows.net/downloads/wp7odatamovies.zip
While I was giving my OData talk, someone asked about consuming OData on the WP7 phone. I had done this on the CTP earlier, but hadn’t tried it during the beta. So I figured I’d look into it today. While this is still pretty easy to do, the tooling still isn’t in place. This means that you can’t simply do an “Add Service Reference” to a Windows Phone 7 project. Instead you have to follow these steps:
- Download the Windows Phone 7 OData Library here.
- Unzip the Windows Phone 7 OData Library to get access to the reference.
- In your project, add a reference to the System.Data.Services.Client.dll assembly from the .zip file.
- Create the proxy classes by using the .NET 4.0’s DataSvcUtil.exe (located in the %windir%\Microsoft.NET\Framework\v4.0.30319 directory). See the example below:
%windir%\Microsoft.NET\Framework\v4.0.30319\DataSvcUtil.exe
/uri:http://odata.netflix.com/Catalog/
/out:NetflixModel.cs
/Version:2.0
/DataServiceCollection
- Include this new proxy class (containing the context object and the data classes) in your WP7 application.
- Use the class to call out to the service as necessary:
NetflixCatalog ctx =
new NetflixCatalog(new Uri("http://odata.netflix.com/Catalog/"));
var qry = from p in ctx.People
.Expand("TitlesDirected")
where p.Name == "Stanley Kubrick"
select p;
// Create DataService query since we're not
// using a DataServiceCollection
var dsQry = (DataServiceQuery<Person>)qry;
dsQry.BeginExecute(r =>
{
try
{
var result = dsQry.EndExecute(r).FirstOrDefault();
if (result != null)
{
Deployment.Current.Dispatcher.BeginInvoke(() =>
{
Titles = result.TitlesDirected;
});
}
}
catch (Exception ex)
{
MessageBox.Show(ex.Message);
}
}, null);
Note that you need to use a Dispatcher as this call is *not* guaranteed to be called on the UI thread. This was the one piece that was unexpected. Once I marshaled the update to the UI thread, all worked perfectly.
Here’s the example: download
