Thanks for visiting my blog!
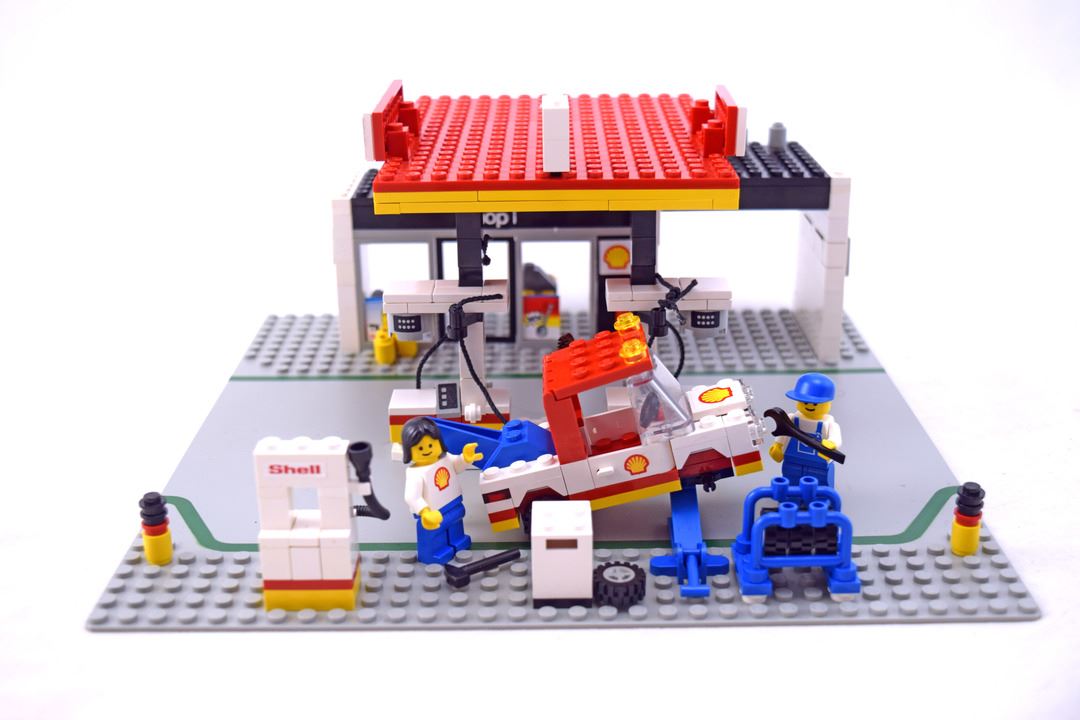
In my spare time, I’ve been working on a micro-services example to try and make a minimum viable micro-service using ASP.NET Core. To make things that much harder, I’ve also decided to use Vue 3 for the front end. In for a penny, in for a pound.
After spending the last month with Vue 3 (or so), I’ve come away with some opinions that I thought I’d share. Some of these are because of the lack of support for Vue 3 for some of the common libraries I used, but in many ways it’s a love letter to some of the features I really love. Her we go…
The Ecosystem
Developing on a Beta can be difficult. Lots of time there are inconsistencies between different versions of packages that are in different states. I haven’t found this to be a particular problem with the Vue ecosystem.
For example, I’m using:
Vue 3 Beta 15
Vue Routing 4 Alpha 13
Vuex 4 Beta 2
They are seem to be in a consistent state most days. The compatibility with other libraries isn’t so simple. One case where this really hurt me was using VeeValidate and Vuelidate libraries (for validating forms) both didn’t work well with Vue 3 so I had to cruft up my own (temporary) solution. If you’re heavily reliant on libraries that are waiting for Vue 3 to release, you might have issues in this early phase.
Composition API
At first, I was concerned about the new Composition API. I had gotten comfortable with the Options API but I was wrong. I really like the new API now that I’ve really had time to dig in.
If you’re not as familiar with it, essentially it’s a way to setup your vue object (e.g. view) with a method that is called instead of a JavaScript object.
The Options API relies on Vue exposing parts of the object to methods in the Vue object. For example:
In the Options API is responsible for making sure the ‘this’ pointer contains all the data in the data object and all the methods in the Vue object. Lots of magic happening here.
In the Composition API, all of this happens with JavaScript closures:
In this case, the fact that name is constructed inside the ‘setup’ function, you get function level closures with the onClick and for returning the data.
The other feature I really like is to actually compose the component from multiple files. Because it’s just a closure, you can just import it from another file if you don’t want to make one huge component file. This was a problem with the Options API as it was more difficult to separate the different parts of the Options object into multiple files. It’s simplified my code.
Vuex in Vue 3
There are lots of places where people are saying the new reactivity wrappers in Vue 3 make Vuex unnecessary, though I like the way that it is structured so I am using it here with little change.
One thing that I had to get used to was to not have access to mapState/mapMethods/etc. from Vuex. But once I understood how the Composition API, I realized that I didn’t really want to use them. Instead, I wanted to just use the store. For example:
Since we might want store objects, we can use closures to get at them. If we just used mapState/mapActions/etc., we’d lose the ability to use them in a closure. In this way, the code becomes less magic, and simpler.
New Feature Use
I also wanted to highlight two smallish features in Vue 3 that I really love.
The first one is watchEffect. While this is similar to a watch, watchEffect can be fired whenever state changes and doesn’t require a specific object to change. For example:
This forces my object to validate itself when any changes in the view happen. This allows for fewer one-off watches as this is affected when the effect changes in a view. Very cool.
Secondly, I’d point out Teleport. This is a feature that React called Portals. The idea is essentially saying that this component has markup that it is responsible for, but render it somewhere else. For example:
In this example, my main Vue component has the top-level menu, but I ‘teleport’ it to the page’s main menu section (which is outside the Vue component). This allows you to have markup that is rendered outside the component which is pretty cool.
You can look at the Vue 3 app I built inside my micro-service example here:
https://github.com/shawnwildermuth/TicketScalper/tree/master/src/TicketScalper.Web/client
Let me know what you’re enjoying about Vue 3 (or just Vue 3 features that you’re using in Vue 2).
