Thanks for visiting my blog! See more about me here: About Me
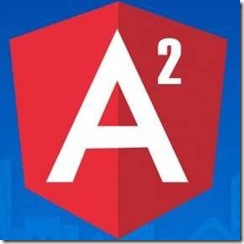
My challenge was to get Angular and ASP.NET Core to work together. I like the idea about Angular-CLI doing all the boilerplate since setup is a bit of a headache. Should be an easy win-win. Well…
UPDATE: Forgot about turning TypeScript off in the project. See Below.
There are a lot of good tutorials and blog posts on using the CLI so I’m just going to talk about how I got Angular and ASP.NET Core 2 working together *in VS2017*.
Before I moved the project into Visual Studio, I needed to tell the project that I’m handling the TypeScript myself. To do that I add two lines to the csproj file:
<Project Sdk="Microsoft.NET.Sdk.Web">
<PropertyGroup>
<TargetFramework>netcoreapp2.0</TargetFramework>
<TypeScriptToolsVersion>latest</TypeScriptToolsVersion>
<TypeScriptCompileBlocked>true</TypeScriptCompileBlocked>
</PropertyGroup>
...
Make sure that the TypeScript version is latest (so that intellisense works right) and make sure you block the compilation of TypeScript; Angular-CLI will do that for us.
I deconstructed the Angular project to live in the ASP.NET Core project (so that the following files ended up in the root of the project):
package.json
tsconfig.json
.angular-cli.json
All these files are needed to get angular working (the last one is the configuration for the actual cli for your project). Then I took the entire src directory (the one with all the .ts files) and put it in the root of the project (but I named it “ClientApp” to make it clearer what it is). Here’s what that looks like:
At this point VS2017 (Preview2) was really unresponsive. It was getting bogged down by the npm stuff and I didn’t know why. So I opened the Output window for npm/bower and saw a ton of errors and failures to load the npm dependencies. Hrmph!
The errors were about needing a newer version of Node and NPM on my machine. I knew I had upgraded so I had these versions already installed on my machine:
But the errors said I wasn’t using these versions. Investigating a usual issue with Visual Studio, I right clicked the** ‘npm’** folder to configure the web tools:
And I could see that it was using the VS installed versions first:
Putting $(PATH) should fix it but it didn’t. What I didn’t realize is that it was using the PATH from the VS Command-line, not the machine-level PATH (which had been set when I updated npm and node).
To fix this I manually added the folders for npm and node. I am sure there is a better fix, but I had to get to work ; )
Now when I restored, all was well!
The only real oddity was that to do the development I needed to launch dotnet in watch mode and Angular-CLI in watch mode. That way I could just change code and the server and client code would be auto-built as I needed. To do this I wrote a tiny little .cmd file:
start ng build --watch
start dotnet watch run
That way I could run both watches and do about my business. This is working pretty well. Anyone have any improvements or know a way to integrate this with launchsettings.json? I don’t want to actually debug necessarily in VS but I like the flow of it so far
