Thanks for visiting my blog! See more about me here: About Me
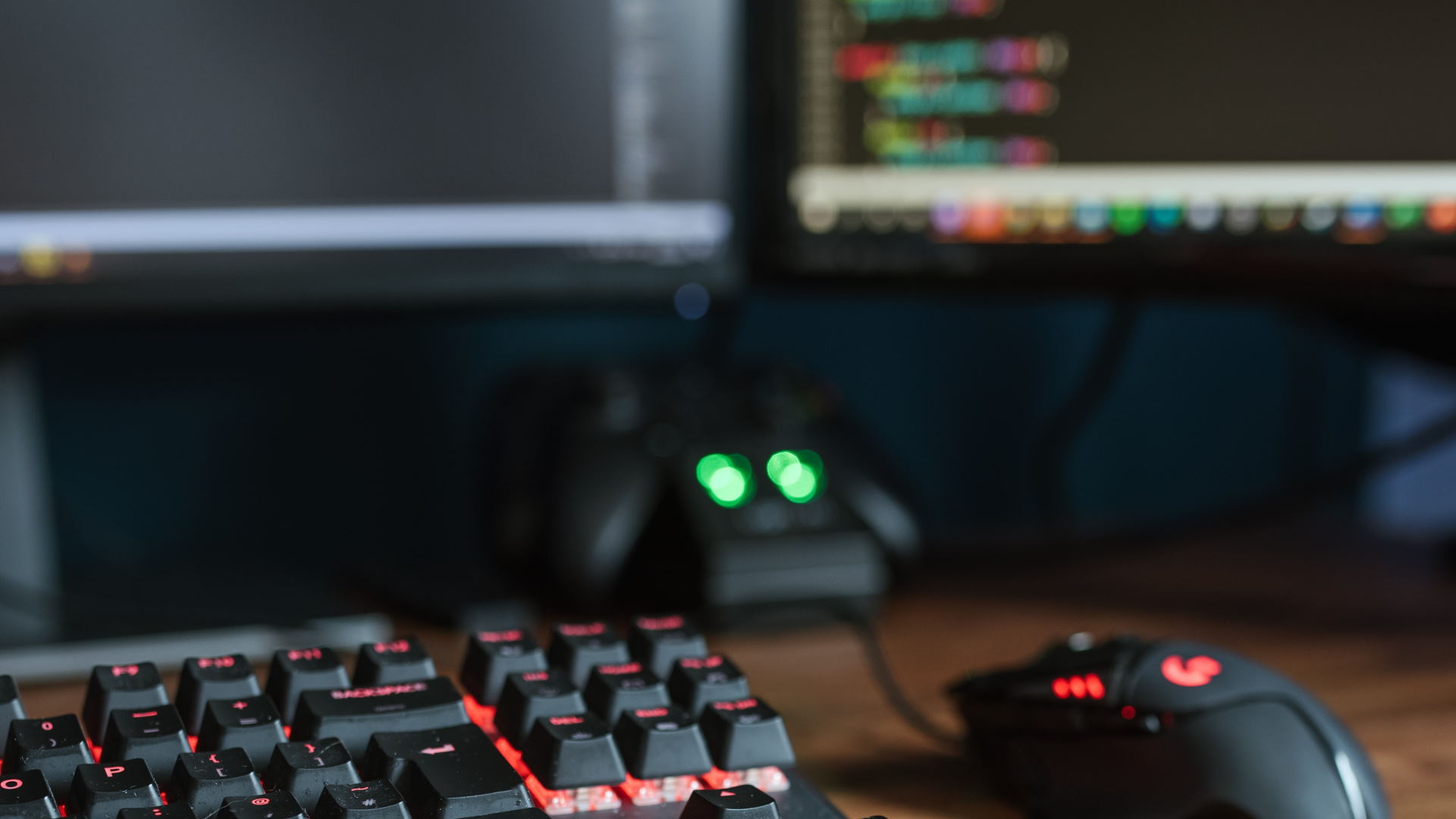
Part 1: Coupling Objects
I have been having a conversation about coupling of objects at the interface level. While I am not a fan of coupling objects together, I would like to be able to shortcut some factory code to make the interface be more intuitive. For example, here is code that decouples the interface:
class Foo
{
// ...
Guid _barID;
public Guid BarID
{
get { return _barID; }
}
}
Foo foo = new Foo();
Bar bar = Bar.GetBar(foo.BarID);
Whereas I don’t want to tie the objects together, but simply call the factory (the GetBar method) within the Foo class to make getting objects easier and more intuitive. For example:
class Foo
{
// ...
Guid _barID;
public Guid BarID
{
get { return _barID; }
}
public Bar GetBar()
{
return Bar.GetBar(_barID);
}
}
Foo foo = new Foo();
Bar bar = foo.GetBar();
Not much different, but it makes the interface a bit clearer about how to get a Bar from Foo. This assumes some information about how assemblies are built. This does not work well if you have circular references in different assemblies. But in most of the project I have worked on, we use larger assemblies so this happens much more infrequently.
Part 2: Assembly Loading
As I was discussing it, the topic came up of which is better, more small assemblies, or fewer large assemblies. The argument comes down to this:
It is my memory (though possibly flawed) that larger assembies really don’t worry about loading lots of code because the real code loaded in the JIT’d code. JIT’d code is certainly created as necessary on a method by method basis. The part my memory is more unsure about is whether all the IL or just the Metadata is read into memory. If all the IL is read in, then the smaller assemblies position has a point.
Any feedback (whether as comments or e-mailed to me directly) is welcomed.
