Thanks for visiting my blog! See more about me here: About Me
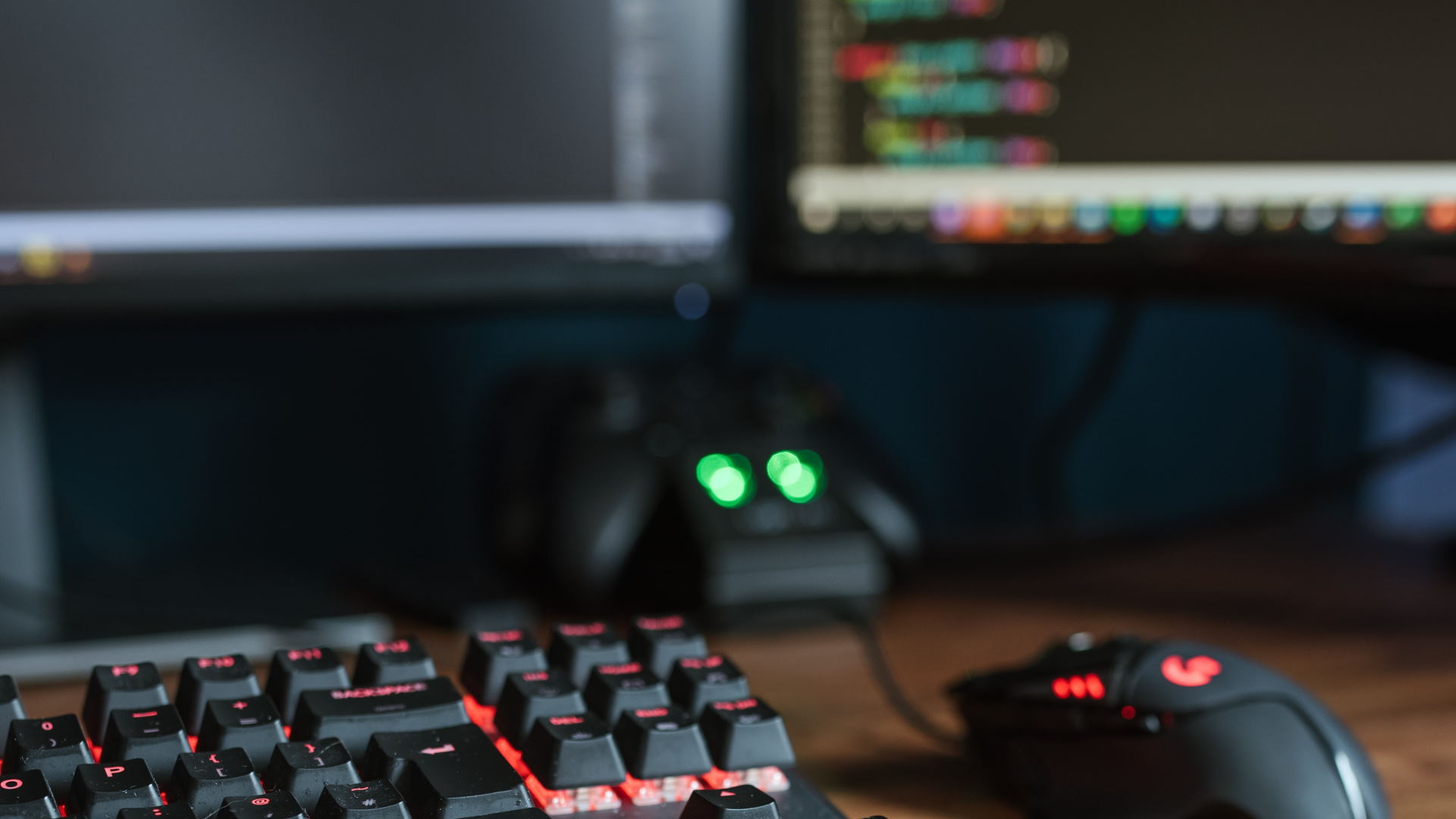
But when I moved the code over, I realized that the old, reliable Cache object was missing. Luckily I found it and like much of ASP.NET Core, adding it was simple and consistent. Let me show you.
First thing was to see how caching was being handled. Instead of having different caches, ASP.NET Core has an abstraction around caching and then you can support different types of caches. For me, it was a simple memory cache I wanted so I needed two Nuget packages:
// project.json
{
...
"dependencies": {
...
"Microsoft.Extensions.Caching.Abstractions": "1.0.0-rc1-final",
"Microsoft.Extensions.Caching.Memory": "1.0.0-rc1-final",
...
Once I had the packages installed, I had to add caching to the service container:
public void ConfigureServices(IServiceCollection svcs)
{
...
// Add Caching Support
svcs.AddCaching();
// Add MVC to the container
svcs.AddMvc()
.AddJsonOptions(opts =>
{
opts.SerializerSettings.ContractResolver = new CamelCasePropertyNamesContractResolver();
});
}
Once caching is available, then in the controller, I can inject an IMemoryCache object:
[Route("")]
public class RootController : Controller
{
private IMemoryCache _memoryCache;
public RootController(IMemoryCache memoryCache)
{
_memoryCache = memoryCache;
}
Finally, I’ve done the wire-up so I can use it in my action:
[HttpGet("fromcache")]
public IActionResult CachedString()
{
var CACHEKEY = "__STRING_TO_CACHE";
string cached;
if (!_memoryCache.TryGetValue(CACHEKEY, out cached))
{
// Get the string to be cached
// TODO
// Decide how to cache it
var opts = new MemoryCacheEntryOptions()
{
SlidingExpiration = TimeSpan.FromHours(2)
};
// Store it in cache
_memoryCache.Set(CACHEKEY, cached, opts);
}
return View(cached);
}
I used TryGetValue to see if the value was already cached, and then if not, just added it to the cache. The options object allows you to set caching options like priority, sliding or absolute expiration.
Hope this helps you if you need caching!
